Unity スティック入力で選択できる選択肢を実装する
Xboxコントローラで遊ぶゲームを作ると、タイトル画面でボタンを選択したいですよね。
簡単にそれを実装する方法(著者がよくやる手法)を紹介します。
Unity Ver.2019.2.21f1
↓今回やること↓
スティック入力で選択できる選択肢の実装。
— わかです (@wakaGameStudio) 2020年8月14日
めちゃ簡単。 pic.twitter.com/T8mCx3GvEg
①UIを作成
- うまく作れば縦横組み合わせて作れるのですが、今回は縦方向のみです。
②Project欄にコンテンツを作成
- imgSelAC:作成したImageすべてにアタッチするAnimatorController
- Select:選択中に再生するAnimator
- Standby:待機中(非選択時)に再生するAnimator
- SelManagerScript:スティック入力や選択後の遷移を管理するスクリプト
③Animatorの設定
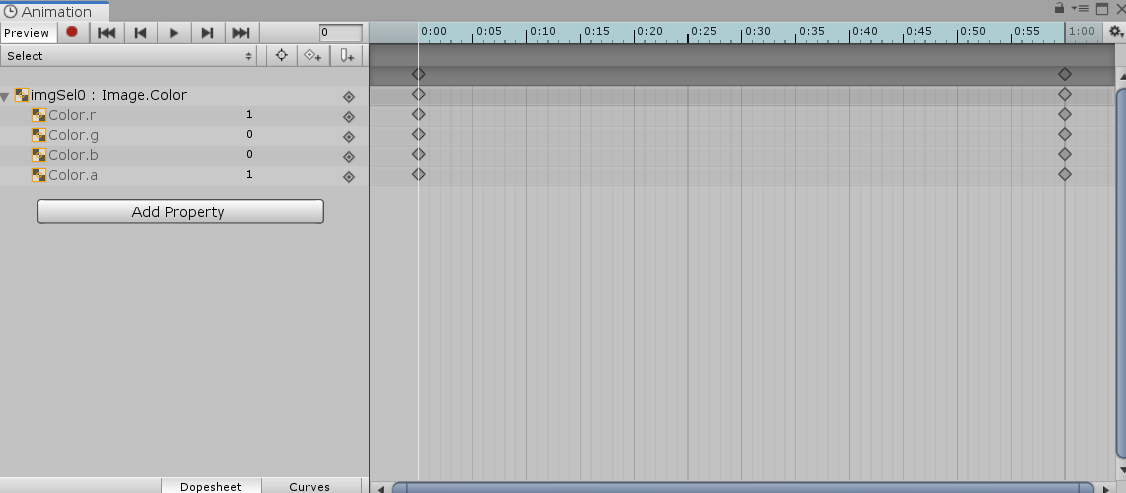
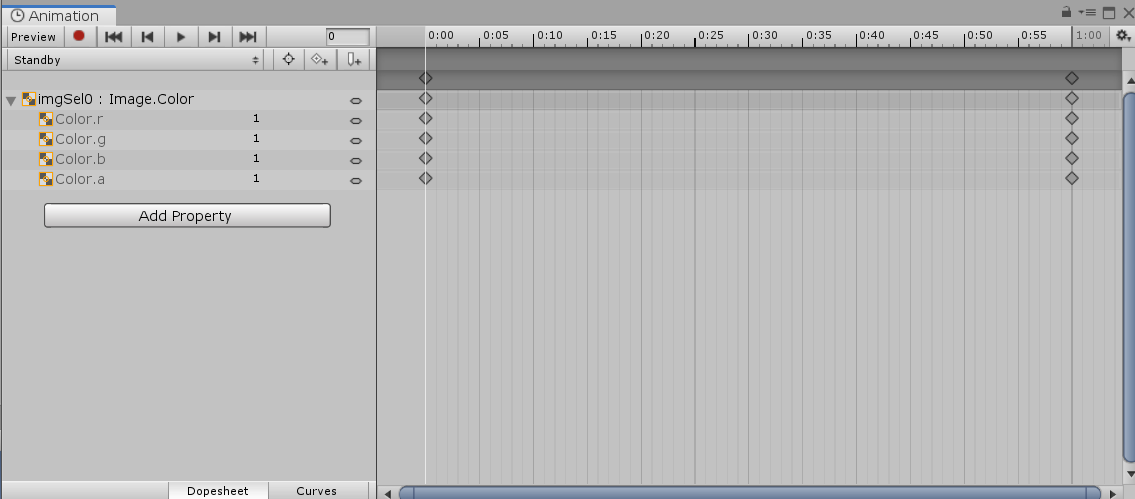
- 今回は、選択時は赤色、非選択時は白にしています。
- Inspector欄でLoop Timeにチェックを入れておきましょう。
④AnimatorControllerの設定
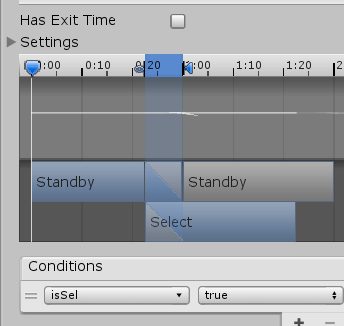

- ParameterにBoolを追加。
- 追加したBoolがtrueなら選択中、falseなら非選択になるように設定。
- HasExitTimeのチェックを外す。
⑤Scriptの中身を書く
using System.Collections; using System.Collections.Generic; using UnityEngine; public class SelManagerScript : MonoBehaviour { //イメージにアタッチしたアニメーターコントローラ [SerializeField] private Animator[] animator; //連続入力回避用フラグ private bool isSel; //選択中番号 private int selCnt; private void Start() { //変数初期化 selCnt = 0; animator[selCnt].SetBool("isSel", true); } private void Update() { //スティックの入力値を格納 float input = Input.GetAxis("Vertical"); //スティックの入力チェック if (input <= -0.5f && !isSel) { isSel = true; animator[selCnt].SetBool("isSel", false); ++selCnt; if (selCnt >= animator.Length) { selCnt = 0; } animator[selCnt].SetBool("isSel", true); } if (input >= 0.5f && !isSel) { isSel = true; animator[selCnt].SetBool("isSel", false); --selCnt; if (selCnt < 0) { selCnt = animator.Length - 1; } animator[selCnt].SetBool("isSel", true); } //長押し回避 if (input >= -0.1f && input <= 0.1f) { isSel = false; } //決定 if (Input.GetKeyDown(KeyCode.Return)) { switch (selCnt) { case 0: print("0を選択"); break; case 1: print("1を選択"); break; case 2: print("2を選択"); break; default: break; } } } }
忘れずにSerializeFieldで出した分をDandDで設定しておきます。
Console欄にご注目。。。
スティック入力で選択できる選択肢の実装。
— わかです (@wakaGameStudio) 2020年8月14日
めちゃ簡単。 pic.twitter.com/T8mCx3GvEg